Gatsby tip on running multiple queries (GraphQL aliases)
1 min read
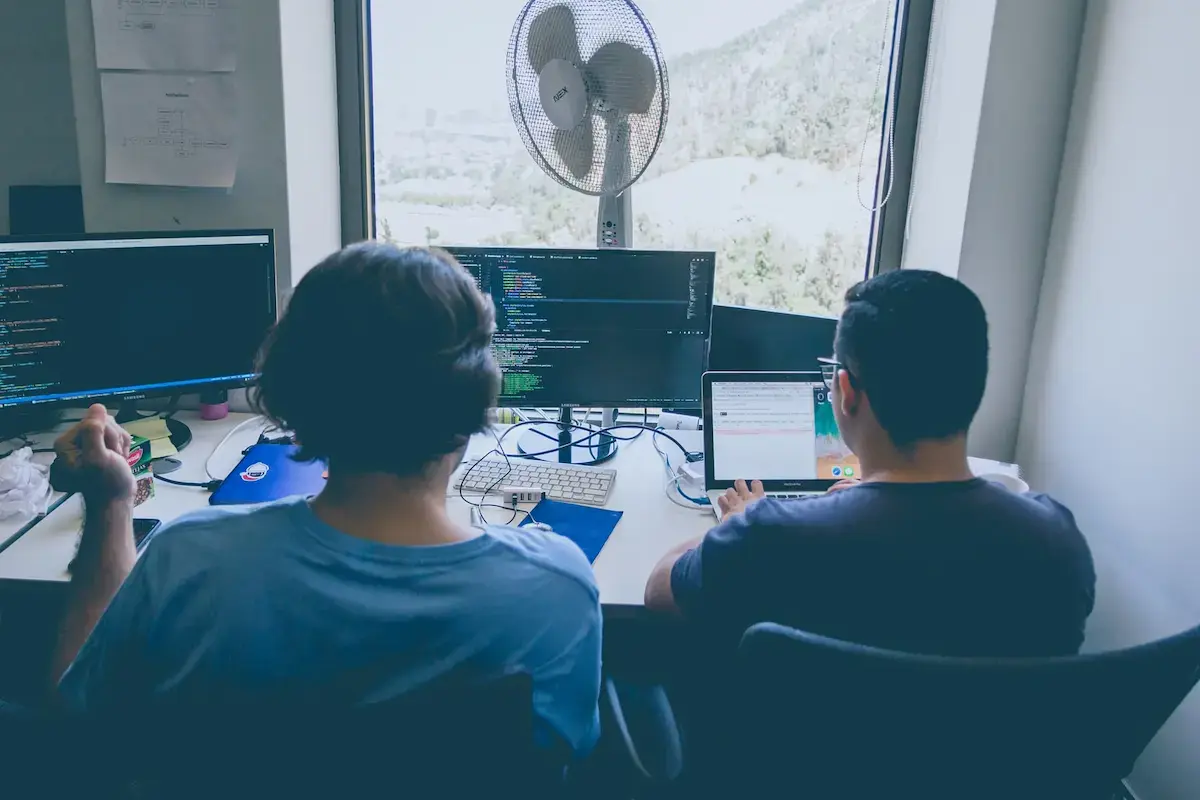
Say you want to fetch specific data in one page based on an argument or a condition which can't be run using one query as you can't query the same field with different condition or argument. One way of doing that by using GraphQL aliases which you can use to rename the returned dataset to anything you want.
Example
GraphQL Alias
1export const query = graphql`2query {3post: allMarkdownRemark(4limit: 35sort: { order: DESC, fields: [frontmatter___date] }6filter: { frontmatter: { type: { ne: "portfolio" } } }7) {8edges {9node {10timeToRead11frontmatter {12title13path14date(formatString: "DD MMMM YYYY")15summary16images17tags18type19}20}21}22}23portfolio: allMarkdownRemark(24sort: { order: DESC, fields: [frontmatter___date] }25filter: { frontmatter: { type: { eq: "portfolio" } } }26) {27edges {28node {29frontmatter {30title31path32images33tags34type35}36}37}38}39siteMetaData: site {40siteMetadata {41title42}43}44}45`;
Looking at the above example, we can see the query I made will return multiple datasets by giving it an alias which allowed me to run multiple queries with different arguments and conditions to get the specific data object I needed as you can see in the screenshot.
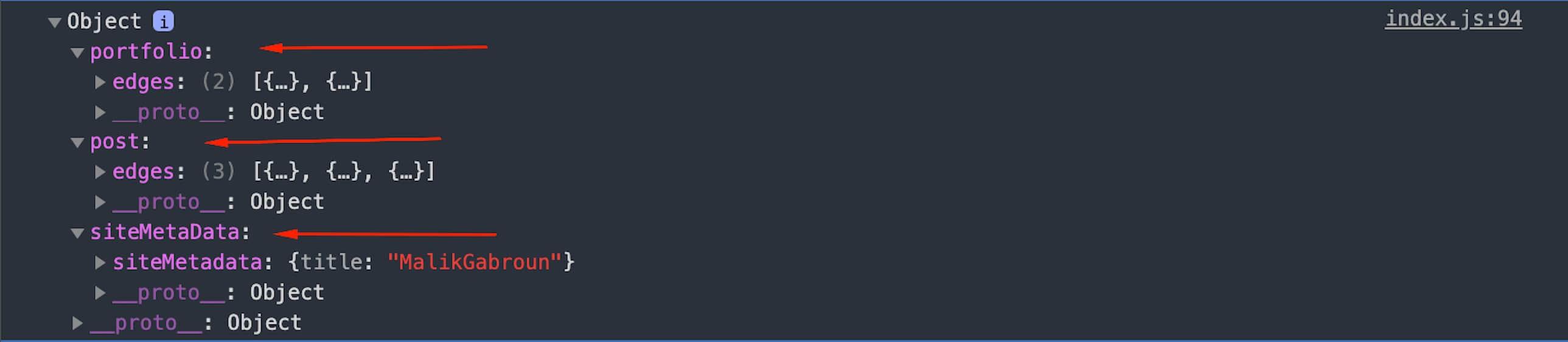