Programmatic Redirects in Gatsby
3 min read
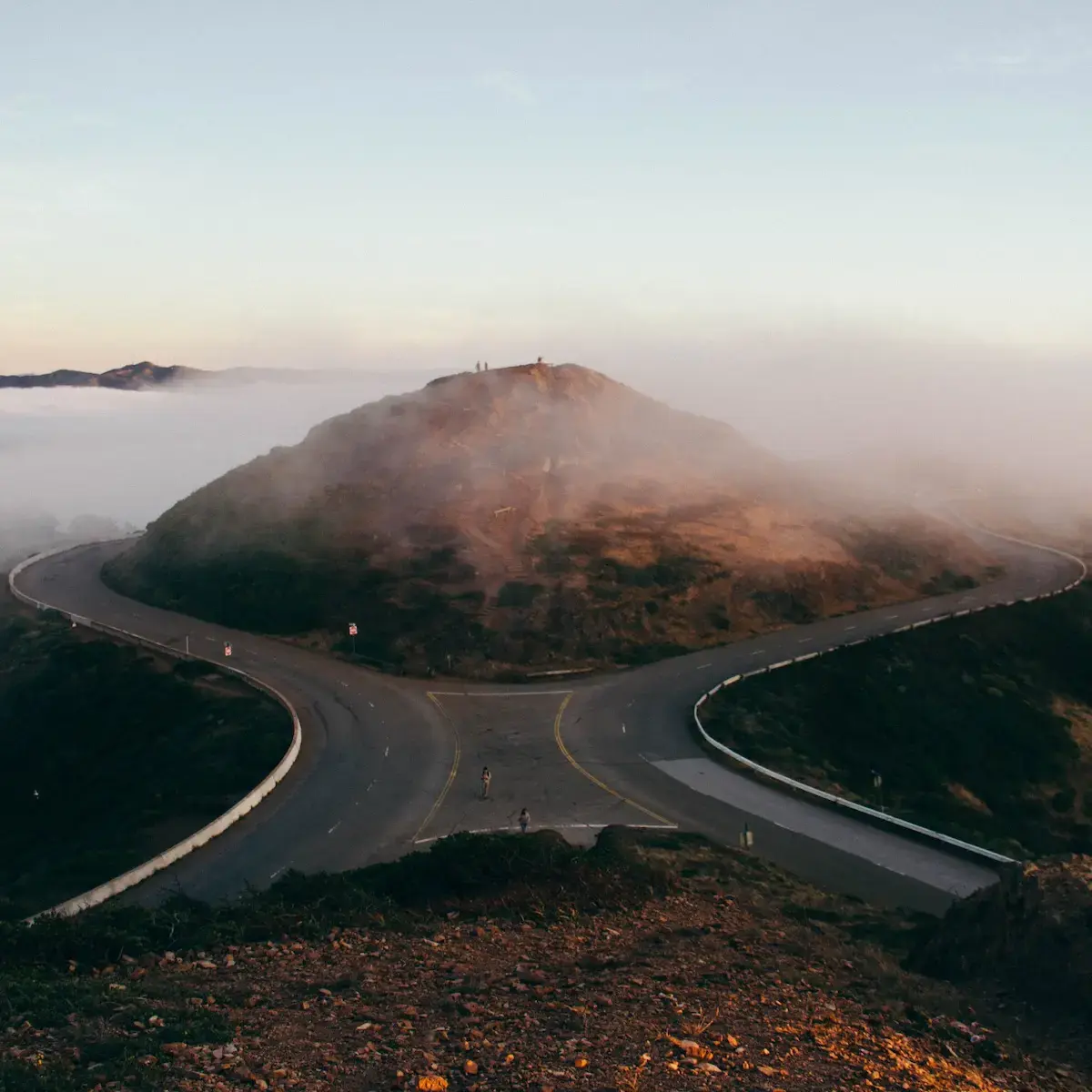
Say we wanted to migrate our posts’ route from one route to another in our site but we do not want to go through the troubles of updating it everywhere else where the URL has been shared. As we want to avoid the issues of 404 pages if the user has visited the old URL route or in case these old routes have been indexed in Google.
In order to solve this issue, we will need to look into how we can redirect users programmatically to the new URL.
So in this post, we will be looking into how we can add redirects programmatically to our site especially if you are using Netlify. There are 2 types of redirects
1. Using Gatsby createRedirect
Gatsby API offers us a variety of utility functions that can be used to extend how gatsby works which can be configured inside gatsby-node.js
, these instructions will be done at build time.
The one in particular that we are interested in and going to use it is createRedirect
function which as the name suggest going to create a redirect from one page to another.
Installation
Additionality, we need to keep in mind that server redirects doest work out of the box so we need to install a plugin to integrate redirect with the hosting service.
npm i gatsby-plugin-netlify
Alternatively, if your choice of hosting service doesn’t have a plugin in gatsby, you can use a meta redirect plugin to generate meta redirect HTML files.
npm i gatsby-plugin-meta-redirect
And then inside gatsby-config.js
we add the plugin to our plugins array
plugins: [`gatsby-plugin-netlify`];
Configuration
To create the redirects we need to update createPages function in gatsby-node.js
which is going to be run once during the process of page creation.
Inside the function, we call createRedirect
method which takes an options object that includes a couple of properties
Example
in the example below, we can see how we can destruct createRedirect from actions object and call it to create the redirects
gatsby-node.js
1exports.createPages = async ({ actions, graphql, reporter }) => {2const { createRedirect } = actions;3const result = await graphql(4`5query {6allMdx {7nodes {8frontmatter {9path10images11type12redirects13}14}15}16}17`18);19if (result.errors) {20reporter.panic("failed to create posts ", result.errors);21}22const pages = result.data.allMdx.nodes;23pages.forEach((page) => {24const { redirects } = page.frontmatter;25if (redirects) {26createRedirect({27fromPath: redirects[0],28toPath: redirects[1],29redirectInBrowser: true,30isPermanent: true,31});32}33actions.createPage({34path: page.frontmatter.path,35component: require.resolve("./src/templates/postTemplate.js"),36context: {37pathSlug: page.frontmatter.path,38image: `resources/${page.frontmatter.images}`,39},40});41});42};
While looping through the results from the GraphQL query, we can check if the page has frontmatter redirects property which is going to be an array that looks like the following
redirects: ['/gatsby-create-pages-with-mdx',
'/blog/gatsby-create-pages-with-mdx']
If it does contain the redirects array, then it is going to create a redirect for that specific page to redirect visiting users to the new path specified. We can check out the available properties that createRedict offers in the Gatsby docs
createRedirect({
fromPath: redirects[0],
toPath: redirects[1],
redirectInBrowser: true,
isPermanent: true,
});
This will allow us to create redirects for static generated sites without the need to mess with routing in the redirects file.
2. Using Netlify _redirects File
We can add all of our redirects manually inside _redirects
file. In order to make sure that we are following the redirect syntax that Netlify uses, we can check it in the docs and test the syntax in the playground they offer here.
Example
In the following below we can take a look at the example of redirects in their playground.
/verifying-gatsby-in-search-console /blog/verifying-gatsby-in-search-console
Which will result in the following
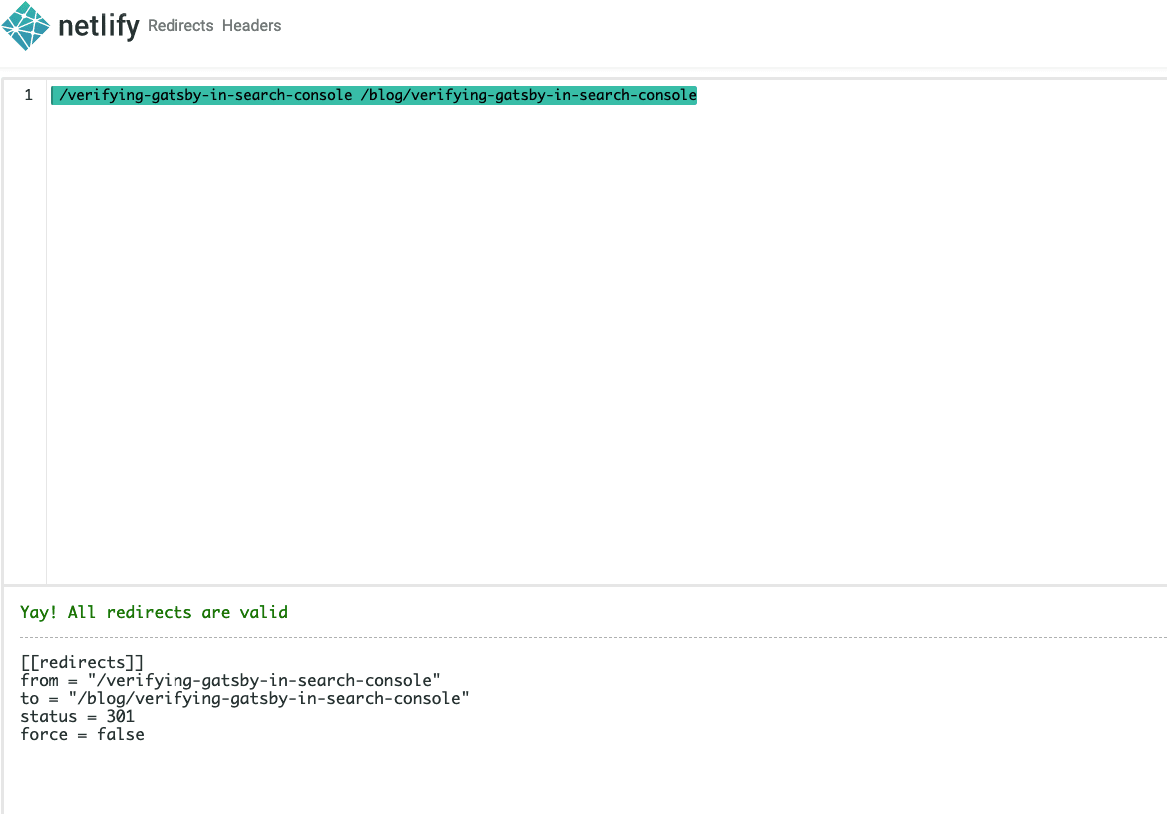
Conclusion
In order to do redirects in gatsby, we have the options of either using _redirects file or following the gatsby way of using createRedirect utility inside gatsby-node.js.